Take values from the user and compute sum, subtraction, multiplication,
division and exponent of value of the variables.
<!DOCTYPE html>
<html>
<head>
<title>Simple Calculator In PHP </title>
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container" style="margin-top: 50px">
<form method="post" >
<input name="number1" type="text" class="form-control" style="width: 150px; display: inline" />
<select name="operation">
<option value="plus">Plus</option>
<option value="minus">Minus</option>
<option value="multiply">Multiply</option>
<option value="divided by">Devide</option>
<option value="exponent">Exponent</option>
</select>
<input name="number2" type="text" class="form-control" style="width: 150px; display: inline" />
<input name="submit" type="submit" value="Calculate" class="btn btn-primary" />
</form>
<?php
if(isset($_POST['submit']))
{
if(is_numeric($_POST['number1']) && is_numeric($_POST['number2']))
{
if($_POST['operation'] == 'plus')
{
$total = $_POST['number1'] + $_POST['number2'];
}
if($_POST['operation'] == 'minus')
{
$total = $_POST['number1'] - $_POST['number2'];
}
if($_POST['operation'] == 'multiply')
{
$total = $_POST['number1'] * $_POST['number2'];
}
if($_POST['operation'] == 'divided by')
{
$total = $_POST['number1'] / $_POST['number2'];
}
if($_POST['operation'] == 'exponent')
{
$a = $_POST['number1'];
$b = $_POST['number2'];
$total = 1;
for ($i=0; $i < $b; $i++) {
$total = $total * $a;
}
// $total = $_POST['number1'] / $_POST['number2'];
}
echo "<h1>{$_POST['number1']} {$_POST['operation']} {$_POST['number2']} equals : {$total}</h1>";
} else {
echo 'Numeric values are required';
}
}
?>
</div>
</body>
</html>
Output
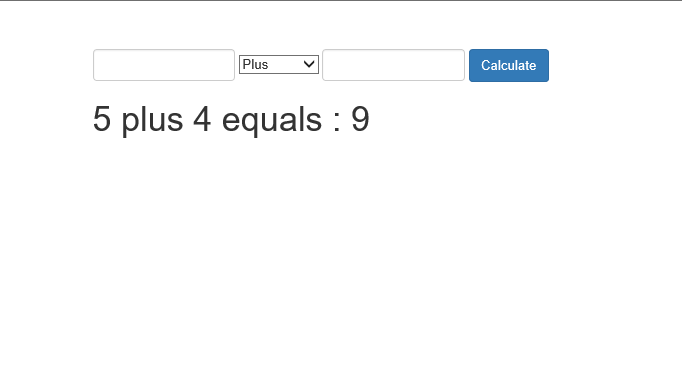
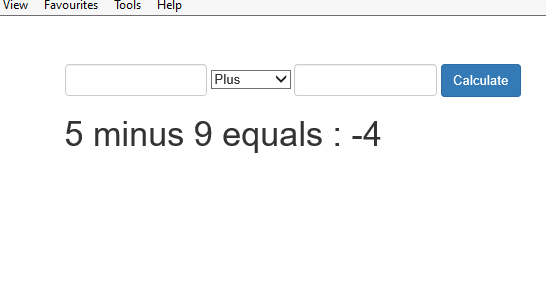
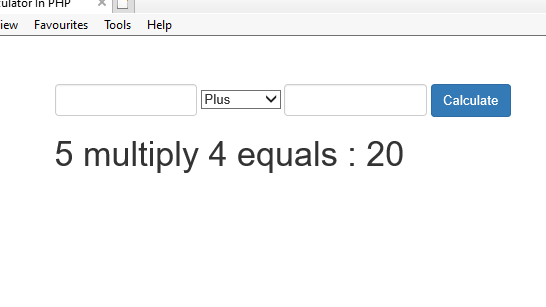
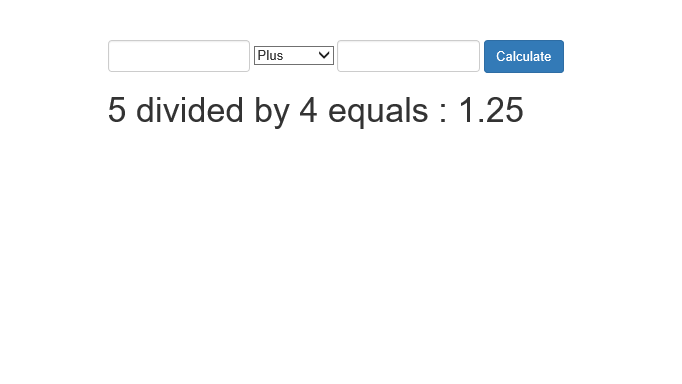
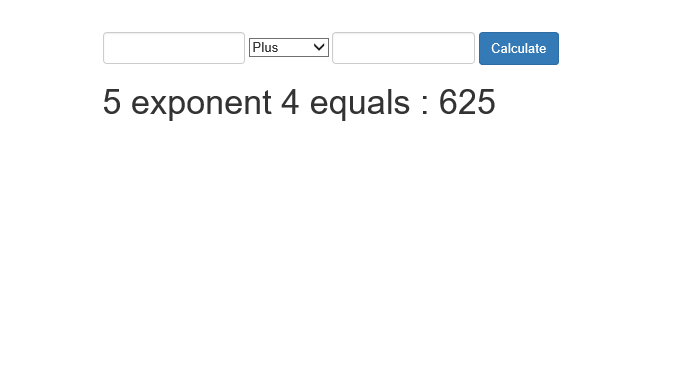